BLOG/Technology
Responsive Javascript Part 2

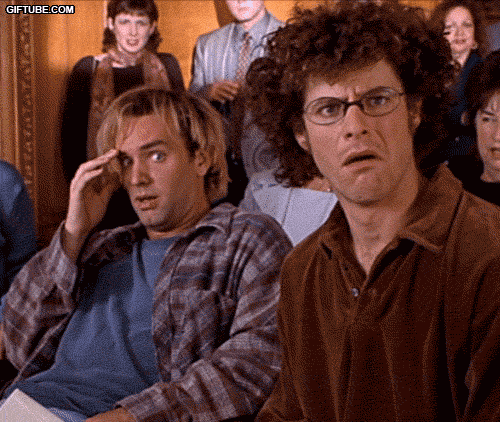
Mark up
<img src="http://lorempixel.com/400/200" class="test" />var imgHeight = $('.test').height(); var imgWidth = $('.test').width(); if( $(window).width() >= 958){ $('.test').mouseenter(function(){ $(this).width(imgWidth * 2).height(imgHeight * 2); }); $('.test').mouseleave(function(){ $(this).width(imgWidth).height(imgHeight); }); }else{ $('.test').click(function(){ if( $(this).width == imgWidth || $(this).height() == imgHeight){ $(this).width(imgWidth * 2).height(imgHeight * 2); }else{ $(this).width(imgWidth).height(imgHeight); } }); }Wrap it in a function and call the function.
var imgHeight = $('.test').height(); var imgWidth = $('.test').width(); function myInteraction(){ if( $(window).width() >= 958){ $('.test').mouseenter(function(){ $(this).width(imgWidth * 2).height(imgHeight * 2); }); $('.test').mouseleave(function(){ $(this).width(imgWidth).height(imgHeight); }); }else{ $('.test').click(function(){ if( $(this).width == imgWidth || $(this).height() == imgHeight ){ $(this).width(imgWidth * 2).height(imgHeight * 2); }else{ $(this).width(imgWidth).height(imgHeight); } }); } } myInteraction();Now wrapping the IF statement in a function like this does essentially the same thing as part 1. But in this case, turning it into a function makes it easier to fire that function repeatedly. Now we want this to trigger every time the window is resized. Doing it this way will force the script to run the function every time the window is resized, the script determines which event to fire base on the width of the browser.
var imgHeight = $('.test').height(); var imgWidth = $('.test').width(); function myInteraction(){ if( $(window).width() >= 958){ $('.test').mouseenter(function(){ $(this).width(imgWidth * 2).height(imgHeight * 2); }); $('.test').mouseleave(function(){ $(this).width(imgWidth).height(imgHeight); }); }else{ $('.test').click(function(){ if( $(this).width == imgWidth || $(this).height() == imgHeight ){ $(this).width(imgWidth * 2).height(imgHeight * 2); }else{ $(this).width(imgWidth).height(imgHeight); } }); } } myInteraction(); $(window).resize(function(){ myInteraction(); });But another issue arises. Now we will have two events stacking, none negating the other. Which is really buggy. To fix this we will need to add some kind of unbind event. Since I'm using jQuery, and .off() event is best.
var imgHeight = $('.test').height(); var imgWidth = $('.test').width(); function myInteraction(){ $('.test').off(); if( $(window).width() >= 958){ $('.test').mouseenter(function(){ $(this).width( imgWidth * 2).height(imgHeight * 2); }); $('.test').mouseleave(function(){ $(this).width( imgWidth).height(imgHeight); }); }else{ $('.test').click(function(){ if( $(this).width == imgWidth || $(this).height() == imgHeight){ $(this).width(imgWidth * 2).height( imgHeight * 2); }else{ $(this).width(imgWidth).height(imgHeight); } }); } } myInteraction(); $(window).resize(function(){ myInteraction(); });The idea here is to remove any events associated every time the function fires then reset the event through the IF statement. BAH-BOOM!!!
More from the
DO Blog
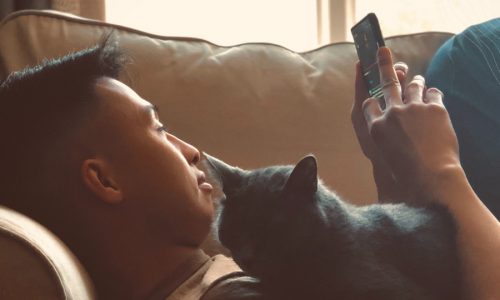
Designing & Building Product Finder Quizzes for eCommer...
Strategy & Planning / December 23, 2020
View Blog Post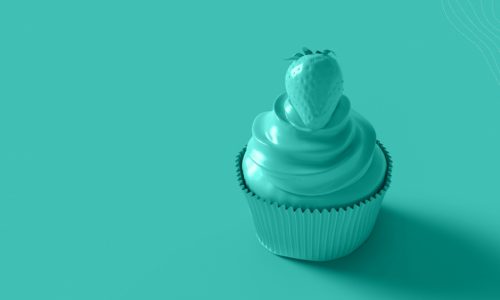
3 Customer Motivation Strategies to Improve Your eCommerce i...
Strategy & Planning / July 27, 2020
View Blog Post